What Does Pass Do In Python
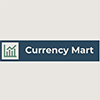
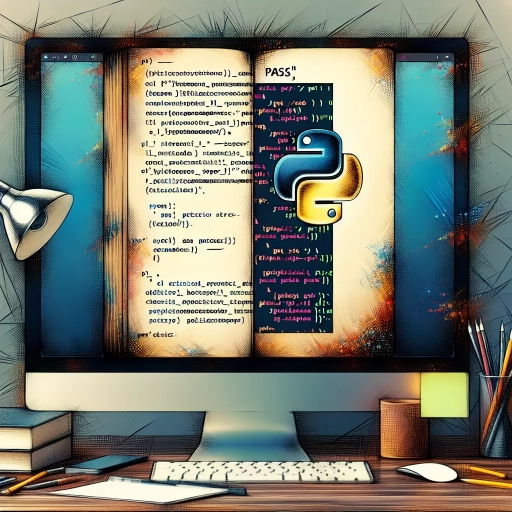
In Python, the `pass` statement is often misunderstood or overlooked, yet it plays a crucial role in various aspects of programming. This article aims to delve into the multifaceted nature of `pass`, starting with a thorough **Understanding of the `pass` Statement in Python**, where we will explore its syntax, purpose, and how it differs from other control structures. We will then examine **Practical Applications of `pass` in Code**, highlighting scenarios where `pass` is essential, such as in placeholder functions, conditional statements, and loops. Finally, we will discuss **Best Practices and Pitfalls with `pass`**, providing insights into how to use `pass` effectively and avoid common mistakes. By the end of this article, you will have a comprehensive understanding of the `pass` statement and its practical uses, beginning with a clear grasp of its fundamental role in Python programming. Let's start by understanding the `pass` statement in Python.
Understanding the `pass` Statement in Python
Understanding the `pass` statement in Python is crucial for any programmer aiming to write efficient and readable code. This article delves into the intricacies of the `pass` statement, exploring its definition and syntax, common use cases, and historical context. The `pass` statement, often misunderstood as a placeholder, plays a significant role in Python programming by allowing developers to create empty code blocks that can be filled later. In the **Definition and Syntax** section, we will examine the precise syntax and how it is used in different contexts. The **Common Use Cases** section will highlight scenarios where `pass` is particularly useful, such as in conditional statements or class definitions. Finally, the **Historical Context** section will provide insight into why `pass` was introduced and its evolution within the Python language. By understanding these aspects, developers can leverage the `pass` statement effectively, enhancing their coding practices. Let's begin by exploring the **Definition and Syntax** of the `pass` statement to grasp its fundamental role in Python programming.
Definition and Syntax
**Definition and Syntax** In Python, the `pass` statement is a placeholder that does nothing when executed. It is used to indicate where a statement is required syntactically but no execution of code is necessary. The primary purpose of `pass` is to avoid syntax errors in situations where a statement is expected but not needed. For instance, when defining a class, function, or loop, Python requires at least one statement within the block. Here, `pass` can be used as a placeholder to satisfy this requirement without performing any action. The syntax of the `pass` statement is straightforward: ```python pass ``` This single keyword can be used alone within any block where a statement is required. For example: ```python class MyClass: pass def my_function(): pass if True: pass ``` In each of these examples, `pass` ensures that the code compiles without errors by providing a necessary statement where none would otherwise exist. The use of `pass` is particularly common during the development phase when you might want to define a structure but delay implementing its details. It allows you to focus on other parts of your code while keeping your program syntactically correct. Additionally, it can serve as a reminder or placeholder for future implementation. For instance, if you are designing a class hierarchy and want to create an abstract base class with methods that will be implemented by subclasses, you might use `pass` in the method definitions: ```python from abc import ABC, abstractmethod class AbstractClassExample(ABC): @abstractmethod def do_something(self): pass ``` Here, `pass` indicates that the method should be implemented by any concrete subclass. In summary, the `pass` statement in Python is a simple yet powerful tool for maintaining syntactic correctness while allowing flexibility in code development. Its definition is clear: it does nothing when executed. Its syntax is minimal: just the keyword `pass`. By understanding and using `pass` effectively, you can streamline your coding process and ensure that your code remains error-free and maintainable.
Common Use Cases
The `pass` statement in Python is often misunderstood but serves several crucial purposes, making it a versatile tool in various coding scenarios. Here are some common use cases where `pass` proves to be particularly useful: 1. **Placeholder for Future Code**: When you're outlining the structure of your program and haven't yet implemented certain sections, `pass` can serve as a placeholder. This allows you to define functions or classes without immediately providing their implementation, ensuring that your code compiles without errors. 2. **Handling Exceptions**: In exception handling, `pass` can be used to ignore exceptions when they occur. For example, if you want to catch an exception but do not need to perform any action upon catching it, `pass` can be used within the `except` block. 3. **Abstract Base Classes**: When defining abstract base classes (ABCs) using the `abc` module, methods that are intended to be overridden by subclasses can be defined with `pass`. This ensures that the method is declared but does not need an immediate implementation. 4. **Conditional Statements**: In conditional statements like `if`, `elif`, and `else`, `pass` can be used when no action is required for a particular condition. This helps maintain the structure of your code without causing syntax errors. 5. **Loops**: Similar to conditional statements, `pass` can be used in loops (`for` and `while`) when no action is needed within the loop body. This is particularly useful during the development phase when you're testing loop logic without performing any operations. 6. **Debugging**: During debugging, `pass` can temporarily replace code blocks that you want to disable or bypass. This allows you to isolate issues by selectively disabling parts of your code without removing them entirely. 7. **Documentation and Readability**: Using `pass` in empty blocks (like empty functions or classes) improves readability by clearly indicating that these blocks are intentionally left blank. This practice helps other developers understand that the absence of code is not an oversight but a deliberate design choice. In summary, the `pass` statement is not just a null operation; it is a deliberate design element that helps in structuring, debugging, and maintaining Python code efficiently. By understanding its various use cases, developers can leverage `pass` to write more robust and maintainable code.
Historical Context
Understanding the historical context of programming languages, particularly Python, is crucial for grasping the nuances of specific statements like `pass`. Python, first released in 1991 by Guido van Rossum, was designed to be a high-level, interpreted language that emphasizes readability and simplicity. The `pass` statement, introduced early in Python's development, serves as a placeholder when a statement is required syntactically but no execution of code is necessary. Historically, the concept of placeholder statements dates back to earlier programming languages. For instance, in languages like C and C++, empty blocks or comments are often used where no action is required. However, these approaches can lead to confusion and make code less readable. In contrast, Python's `pass` statement provides an explicit way to indicate that a block of code is intentionally left empty. The introduction of `pass` aligns with Python's philosophy of clarity and explicitness. It helps developers avoid common pitfalls such as using `None` or `0` as placeholders, which can lead to misunderstandings about the intent of the code. For example, in a class definition where you might want to define a method but not implement it immediately, using `pass` makes it clear that the method is intentionally left empty rather than forgotten. Moreover, the historical evolution of Python has seen significant contributions from its community. The language's design has been influenced by various other languages such as ABC, Modula-3, and C. The inclusion of `pass` reflects this broader context by providing a feature that enhances code readability—a key aspect of Python's design principles. In practical terms, understanding the historical context behind `pass` helps developers appreciate why it exists and how it should be used effectively. For instance, when defining abstract base classes or stubbing out functions for future implementation, `pass` ensures that the code remains syntactically correct while clearly communicating its intent. In summary, the `pass` statement in Python is rooted in the language's historical development and design philosophy. It serves as an essential tool for maintaining code clarity and readability, reflecting Python's commitment to simplicity and explicitness. By understanding this context, developers can better utilize `pass` to write more effective and maintainable code.
Practical Applications of `pass` in Code
The `pass` statement in programming, often overlooked due to its simplicity, holds significant practical value in various coding scenarios. This article delves into three key applications of `pass`, each highlighting its utility in different contexts. First, we explore how `pass` can serve as a placeholder for future code, allowing developers to structure their programs without immediate implementation details. Next, we discuss its role in handling exceptions, where `pass` can be used to ignore specific exceptions or maintain code flow when certain conditions are met. Finally, we examine how `pass` improves code readability by providing a clear indication of intentional no-operation, making the codebase more understandable and maintainable. By understanding these practical applications, developers can leverage the `pass` statement to enhance their coding practices. Let's begin by looking at how `pass` can be used as a placeholder for future code, enabling a more structured approach to software development.
Placeholder for Future Code
In the context of Python programming, the `pass` statement serves as a placeholder for future code, allowing developers to structure their programs without immediately implementing all the details. This is particularly useful during the initial stages of development when the overall architecture and logic of the code are being laid out. Here’s how `pass` can be practically applied: 1. **Skeleton Code**: When creating a new class, function, or loop, you might not have all the implementation details ready. Using `pass` allows you to define the structure without raising a `SyntaxError` for an empty block. For example, if you're designing a class but haven't yet decided on its methods, you can use `pass` to fill in the method bodies temporarily. ```python class MyClass: def my_method(self): pass ``` 2. **Conditional Statements**: In conditional statements like `if`, `elif`, and `else`, you might need to handle different scenarios but haven't figured out the exact implementation yet. Placing a `pass` statement ensures that your code compiles without errors while you decide on the actual logic. ```python if some_condition: pass # To be implemented later else: print("Condition not met") ``` 3. **Exception Handling**: When dealing with exceptions, you might want to catch an exception but not take any action immediately. A `pass` statement can be used within an `except` block to ignore the exception temporarily. ```python try: # Some code that might raise an exception except Exception: pass # Ignore the exception for now ``` 4. **Debugging**: During debugging, you might want to temporarily bypass certain sections of your code. Using `pass` in these sections allows you to skip over them without removing the code entirely. ```python def debug_function(): # Code to be executed during normal operation pass # Bypass this section for debugging purposes ``` 5. **Documentation and Planning**: Sometimes, developers use `pass` as a reminder or placeholder for areas where additional functionality needs to be added later. This helps in maintaining a clear roadmap of what needs to be implemented in future iterations. ```python def future_feature(): """This function will implement a new feature.""" pass # To be implemented in the next sprint ``` In summary, the `pass` statement in Python is a versatile tool that helps developers maintain clean, structured code while allowing them to focus on other aspects of their project without worrying about immediate implementation details. By using `pass`, you ensure that your code remains syntactically correct and ready for future enhancements.
Handling Exceptions
Handling exceptions is a crucial aspect of robust and reliable software development, particularly when discussing the practical applications of the `pass` statement in Python. Exceptions occur when an error happens during the execution of a program, and if not managed properly, they can lead to program crashes or unexpected behavior. Python's exception handling mechanism allows developers to anticipate and manage these errors gracefully. In Python, exceptions are raised using the `raise` keyword and caught using the `try-except` block. The `try` block contains code that might potentially raise an exception, while the `except` block contains code that will be executed if an exception is raised. However, there are scenarios where you might want to temporarily ignore or bypass certain sections of code without causing an error. This is where the `pass` statement comes into play. The `pass` statement in Python is a placeholder that does nothing when executed. It is useful for defining a class, function, or control structure that does nothing but is syntactically required. For instance, if you are writing a `try-except` block and you do not want to handle the exception immediately but still need to maintain the structure, you can use `pass` within the `except` block. This ensures that your code compiles without errors while allowing you to revisit and handle the exception properly later. Here’s an example: ```python try: # Code that might raise an exception x = 1 / 0 except ZeroDivisionError: pass # Temporarily ignoring the exception ``` In this example, the `pass` statement allows the program to continue running without halting due to the division by zero error. However, this should be used judiciously as ignoring exceptions without proper handling can lead to silent failures and make debugging more challenging. Another practical application of `pass` in exception handling is during the development phase. You might have a skeleton of your exception handling structure but not yet have the logic to handle specific exceptions. Using `pass` allows you to keep your code syntactically correct while you focus on other parts of your program. For example: ```python try: # Code that might raise an exception x = 1 / 0 except ZeroDivisionError: pass # Placeholder for future exception handling logic except TypeError: pass # Placeholder for future exception handling logic ``` This approach ensures that your code remains structured and maintainable even as you continue to develop and refine it. In summary, while `pass` itself does not handle exceptions directly, it serves as a valuable tool in maintaining the structure of your exception handling code. By using `pass`, you can ensure that your program remains syntactically correct and functional even when you are in the process of implementing or refining your exception handling logic. This makes it easier to manage complex codebases and ensures that your program behaves predictably under various conditions.
Improving Code Readability
Improving code readability is crucial for maintaining and enhancing software projects over time. It involves several key strategies that make your code easier to understand and navigate. **Consistent Naming Conventions** are essential; using clear, descriptive variable and function names helps other developers quickly grasp the purpose of each piece of code. **Comments** should be used liberally to explain complex logic or non-obvious sections of code, but they should not repeat what the code itself clearly states. **Whitespace** and proper indentation are vital for visual clarity, making it easier to distinguish between different blocks of code. **Modular Code** is another important aspect; breaking down large functions into smaller, more manageable pieces not only improves readability but also makes debugging simpler. **Type Hints** in languages like Python can significantly enhance readability by providing immediate insight into the expected data types of variables and function parameters. Additionally, **Error Handling** should be transparent and well-documented to ensure that any issues are clearly communicated and understood. In the context of Python's `pass` statement, improving readability means using it judiciously. The `pass` statement can serve as a placeholder when you need to define a function or class but do not yet have the implementation details. This helps maintain the structure of your code while avoiding syntax errors. For example, if you are designing a class with methods that will be implemented later, using `pass` in those method definitions keeps your code organized and readable: ```python class MyClass: def method1(self): pass # To be implemented later def method2(self): pass # To be implemented later ``` By doing so, you ensure that your code remains clean and easy to follow even as you continue to develop it. This approach aligns with best practices for maintaining high-quality, readable code that is both efficient and understandable.
Best Practices and Pitfalls with `pass`
When discussing best practices and pitfalls associated with the `pass` statement in programming, it is crucial to approach the topic with a comprehensive understanding of its implications. The `pass` statement, often misunderstood or misused, can significantly impact code readability and maintainability. This article delves into three key areas: **Avoiding Misuse**, **Debugging Techniques**, and **Alternative Solutions**. By understanding how to avoid common misuses of `pass`, developers can prevent unnecessary complexity and errors. Effective debugging techniques involving `pass` can help identify issues more efficiently. Additionally, exploring alternative solutions can provide better ways to achieve the desired functionality without relying on `pass`. By mastering these best practices, developers can write more robust and maintainable code. Let's begin by examining the critical aspect of **Avoiding Misuse**, as it lays the foundation for understanding the broader implications of using `pass` in your code.
Avoiding Misuse
When working with the `pass` statement in Python, it is crucial to avoid its misuse to maintain clear, efficient, and readable code. Here are some best practices and pitfalls to consider: **Best Practices:** 1. **Placeholder Use:** Use `pass` as a placeholder when you need to define a function or class but do not have the implementation details yet. This helps in structuring your code without causing syntax errors. 2. **Conditional Statements:** In conditional statements like `if` or `else`, use `pass` if you do not need to execute any code under certain conditions. For example, `if condition: pass` can be used when no action is required. 3. **Exception Handling:** In exception handling blocks, `pass` can be used if you want to ignore an exception without taking any action. **Pitfalls:** 1. **Unused Code:** Avoid using `pass` in production code as it does nothing and can indicate unfinished or unused code. Regularly review your code to ensure that all `pass` statements are replaced with actual implementations. 2. **Confusion:** Overuse of `pass` can lead to confusion among team members or future maintainers of the codebase. It is essential to comment why `pass` is being used if it is necessary. 3. **Performance Impact:** While `pass` itself does not impact performance significantly, its presence can indicate areas where more efficient or meaningful code could be written, potentially improving overall performance. **Example Usage:** ```python def future_function(): # This function will be implemented later pass if some_condition: # No action needed here pass try: # Some operation except Exception: # Ignore the exception for now pass ``` By following these guidelines, you can ensure that the `pass` statement is used judiciously and does not lead to misunderstandings or inefficiencies in your Python code. Regularly reviewing and updating your codebase will help in maintaining high-quality, readable, and efficient software.
Debugging Techniques
Debugging is an essential part of software development, and mastering various debugging techniques can significantly enhance a developer's productivity and code quality. When discussing best practices and pitfalls, especially in the context of using `pass` in Python, it's crucial to understand how debugging fits into the broader picture of coding. **Systematic Debugging Approach:** 1. **Identify the Problem:** Start by clearly defining the issue. This involves reproducing the error consistently to understand its behavior. 2. **Isolate the Issue:** Narrow down the problematic code segment using techniques like binary search or commenting out sections of code. 3. **Use Debugging Tools:** Leverage built-in tools such as Python's `pdb` module or third-party libraries like `ipdb` for interactive debugging. 4. **Print Statements and Logging:** Insert print statements or use logging to monitor variable values and function calls at critical points. 5. **Unit Tests:** Write unit tests to ensure individual components are functioning correctly before integrating them into larger systems. **Best Practices:** - **Consistent Code Structure:** Maintain a consistent coding style to make it easier to identify where issues might arise. - **Error Handling:** Implement robust error handling mechanisms to catch and handle exceptions gracefully. - **Code Reviews:** Regularly review code with peers to catch potential bugs early. - **Version Control:** Use version control systems like Git to track changes and revert if necessary. **Pitfalls with `pass`:** - **Misuse as Placeholder:** Avoid using `pass` as a placeholder without clear intent. It can lead to confusion about whether the block is intentionally left empty or if it's a placeholder that needs to be filled. - **Overuse:** Refrain from using `pass` excessively, as it can clutter the codebase and obscure meaningful logic. - **Debugging Challenges:** When debugging, empty blocks marked with `pass` can make it difficult to understand where issues are occurring because they do not provide any diagnostic information. **Effective Use of `pass`:** - **Syntax Compliance:** Use `pass` when you need a syntactically valid statement but do not want any execution (e.g., in empty classes or functions). - **Temporary Placeholders:** Use `pass` temporarily during development if you plan to fill in the logic later, but ensure it is clearly commented as such. By combining these debugging techniques with best practices and avoiding common pitfalls associated with using `pass`, developers can write more robust, maintainable, and efficient code. This approach not only helps in identifying and fixing bugs but also enhances overall coding hygiene and readability.
Alternative Solutions
When exploring the nuances of Python's `pass` statement, it's crucial to consider alternative solutions that can achieve similar or better outcomes. The `pass` statement is often used as a placeholder when a statement is required syntactically but no execution of code is necessary. However, there are scenarios where other constructs can be more effective or clearer in intent. ### Alternative Solutions 1. **Using Comments for Placeholders:** Instead of using `pass`, you can use comments to indicate that a block of code is intentionally left empty. For example: ```python if condition: # TODO: Implement this block ``` This approach makes it clear that the block is not yet implemented and avoids the ambiguity that `pass` might introduce. 2. **Raising an Exception:** If a function or method is not yet implemented, raising a `NotImplementedError` can be more informative than using `pass`. This clearly signals to other developers that the functionality is pending. ```python def my_function(): raise NotImplementedError("This function is not yet implemented") ``` 3. **Using Abstract Base Classes:** In object-oriented programming, if you have an abstract method that must be implemented by subclasses, using an abstract base class (ABC) with the `@abstractmethod` decorator is more explicit than using `pass`. ```python from abc import ABC, abstractmethod class BaseClass(ABC): @abstractmethod def my_method(self): pass ``` This ensures that any subclass must implement the method, making the code more robust. 4. **Logging or Debugging Statements:** For debugging purposes, you might want to log a message instead of using `pass`. This can help in understanding the flow of your program. ```python import logging if condition: logging.debug("Condition met but no action taken") ``` 5. **Empty Functions with Docstrings:** If you need to define a function that does nothing but want to provide documentation, you can use an empty function with a docstring. ```python def my_function(): """This function does nothing.""" return None ``` This approach maintains readability and provides context through the docstring. ### Best Practices - **Clarity Over Brevity:** While `pass` is concise, it may not always be clear. Using comments or raising exceptions can make your code more understandable. - **Consistency:** Stick to one approach throughout your project. If you decide to use comments for placeholders, use them consistently. - **Documentation:** Always document your code, especially when using placeholders or abstract methods. This helps other developers understand the intent behind your code. ### Pitfalls - **Ambiguity:** Using `pass` without clear documentation can lead to confusion about whether the block is intentionally empty or if it's a placeholder that needs implementation. - **Silent Failures:** Relying solely on `pass` might mask issues where a block of code should perform some action but does not due to oversight. - **Maintenance:** Over time, placeholders can get forgotten. Regularly reviewing your codebase for such placeholders is essential. By considering these alternative solutions and best practices, you can write more robust, maintainable, and understandable Python code.