What Does == Mean In Python
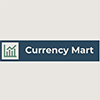
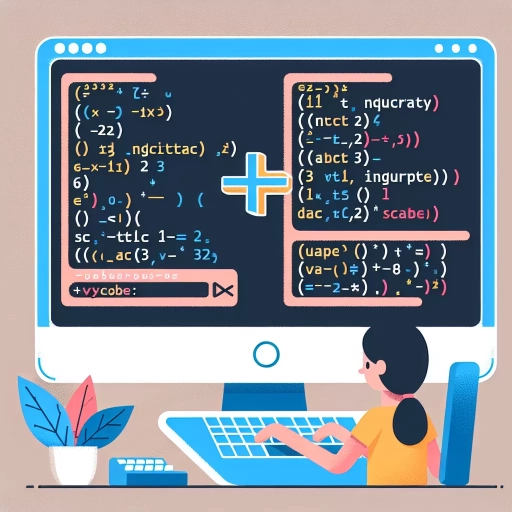
Understanding the += Operator in Python
Understanding the `+=` operator in Python is crucial for any programmer aiming to write efficient and readable code. This operator, often referred to as the augmented assignment operator, simplifies the process of adding a value to a variable and assigning the result back to that variable. To fully grasp its utility, it's essential to delve into three key aspects: **Definition and Syntax**, **Common Use Cases**, and **Example Code Snippets**. First, understanding the **Definition and Syntax** of the `+=` operator will provide a solid foundation. This involves recognizing how it combines addition and assignment in a single statement, which can significantly reduce code redundancy. Next, exploring **Common Use Cases** will highlight scenarios where this operator is particularly beneficial, such as in loops or when dealing with cumulative sums. Finally, examining **Example Code Snippets** will illustrate practical applications, making it easier to integrate this operator into your own projects. By starting with a clear understanding of the **Definition and Syntax**, you'll be well-prepared to appreciate the broader implications and practical applications of the `+=` operator in Python. Let's begin by examining the definition and syntax of this powerful operator.
Definition and Syntax
In the context of understanding the `+=` operator in Python, it is crucial to delve into the broader concepts of definition and syntax. **Definition** refers to the precise explanation or meaning of a term, concept, or symbol within a programming language. In Python, operators like `+=` are defined as shorthand for specific operations that combine assignment and arithmetic or other types of operations. For instance, the `+=` operator is defined as an augmented assignment operator that adds the value on its right to the variable on its left and assigns the result back to that variable. **Syntax**, on the other hand, pertains to the rules governing how symbols, words, and phrases are combined to form valid expressions in a programming language. The syntax of an operator like `+=` includes its placement relative to variables and values, as well as any constraints on its usage. For example, in Python, the syntax for using `+=` involves placing it between a variable and an expression: `variable += expression`. This syntax ensures that the operation is performed correctly and consistently across different contexts. Understanding these definitions and syntax rules is essential for mastering Python programming. When you see `x += y`, you know it means "take the current value of `x`, add `y` to it, and then assign this new value back to `x`." This clarity in definition and adherence to syntax allows developers to write efficient, readable code. Moreover, knowing how operators like `+=` are defined and used syntactically helps in debugging code by identifying potential errors or unexpected behaviors. For instance, consider a simple example where you want to increment a counter by a certain amount repeatedly: ```python counter = 0 increment = 5 counter += increment print(counter) # Output: 5 ``` Here, the definition of `+=` ensures that `counter` is updated correctly by adding `increment` to it. The syntax of placing `+=` between `counter` and `increment` makes this operation clear and concise. In summary, grasping the definitions and syntax of operators such as `+=` in Python enhances your ability to write robust, maintainable code. It allows you to leverage these operators effectively in various programming scenarios, ensuring that your code is both efficient and easy to understand. By understanding these fundamental concepts, you can unlock more advanced features of Python and improve your overall programming skills.
Common Use Cases
When delving into the nuances of Python, understanding the `+=` operator is crucial for effective coding. This operator, known as the augmented assignment operator, is a shorthand for adding a value to a variable and then assigning the result back to that variable. Here are some common use cases that highlight its versatility and utility: **1. Simple Arithmetic Operations:** The `+=` operator is frequently used for simple arithmetic operations where you need to increment a variable by a certain value. For example, if you have a counter variable `count` and you want to increment it by 1 each time an event occurs, you can use `count += 1`. This is more concise than writing `count = count + 1`. **2. String Concatenation:** In Python, the `+=` operator can also be used with strings to concatenate them. If you have a string variable `message` and you want to append another string to it, you can use `message += 'new text'`. This is particularly useful in loops where you might be building a large string incrementally. **3. List Appending:** When working with lists, the `+=` operator allows you to extend the list by appending elements from another list or iterable. For instance, if you have two lists `list1` and `list2`, you can combine them using `list1 += list2`, which is equivalent to using the `extend` method but more concise. **4. Dictionary Updates:** In Python 3.5 and later, the `+=` operator can be used with dictionaries to update their contents. If you have two dictionaries `dict1` and `dict2`, you can update `dict1` with the key-value pairs from `dict2` using `dict1.update(dict2)` or more succinctly with `dict1 |= dict2`. However, for older versions of Python or specific use cases, understanding how `+=` works with other data types is essential. **5. Mathematical Computations:** In more complex mathematical computations, the `+=` operator can simplify code readability. For example, in a loop where you are accumulating sums or products, using `total += value` instead of `total = total + value` makes the code cleaner and easier to understand. **6. Real-world Applications:** In real-world applications such as data processing, machine learning, or web development, the `+=` operator often finds its place in loops where incremental updates are necessary. For instance, in a web scraper script where you are accumulating data from multiple pages, using `data += new_data` ensures that your data collection process remains efficient and readable. Understanding these common use cases for the `+=` operator in Python not only enhances your coding skills but also makes your code more readable and maintainable. By leveraging this operator effectively, you can write more efficient and elegant code that reflects best practices in software development. Whether you are working on simple scripts or complex applications, mastering the `+=` operator is an essential part of becoming proficient in Python programming.
Example Code Snippets
When delving into the nuances of Python, understanding the `+=` operator is crucial for efficient and readable code. This operator, often referred to as the "augmented assignment" operator, simplifies the process of adding a value to a variable and then reassigning it back to that variable. To illustrate its usage and benefits, let's examine some example code snippets. ### Basic Usage ```python x = 5 x += 3 print(x) # Output: 8 ``` In this simple example, `x += 3` is equivalent to `x = x + 3`. The `+=` operator combines these two steps into one, making the code more concise. ### String Concatenation ```python greeting = "Hello, " greeting += "world!" print(greeting) # Output: Hello, world! ``` Here, the `+=` operator is used for string concatenation. It appends "world!" to the end of "Hello, ", resulting in a single string. ### List Extension ```python numbers = [1, 2, 3] numbers += [4, 5, 6] print(numbers) # Output: [1, 2, 3, 4, 5, 6] ``` This snippet demonstrates how `+=` can be used to extend a list by appending another list or iterable. ### Dictionary Update ```python data = {"a": 1, "b": 2} data.update({"c": 3, "d": 4}) data["e"] = data.get("e", 0) + 5 # Using += with dictionary values print(data) # Output: {'a': 1, 'b': 2, 'c': 3, 'd': 4, 'e': 5} ``` While dictionaries do not directly support the `+=` operator for updating values, you can achieve similar functionality by using methods like `.update()` or by manually updating values as shown. ### Real-World Application Consider a scenario where you need to keep track of scores in a game: ```python player_score = 0 player_score += 10 # Score increased by 10 points player_score += 20 # Score increased by another 20 points print(player_score) # Output: 30 ``` Here, the `+=` operator simplifies the process of updating the player's score, making the code easier to read and maintain. ### Performance Considerations While `+=` is generally more readable and convenient, it's worth noting that for large lists or strings, repeated use of `+=` can lead to inefficiencies due to the creation of new objects. For example: ```python large_list = [] for i in range(1000000): large_list += [i] ``` This approach can be slow because it involves creating a new list on each iteration. Instead, using `extend()` or a list comprehension would be more efficient: ```python large_list = [] for i in range(1000000): large_list.extend([i]) # Or using list comprehension large_list = [i for i in range(1000000)] ``` In summary, the `+=` operator in Python is a powerful tool that enhances code readability and efficiency by combining assignment and addition operations into one step. Through various examples, we've seen its application in different contexts such as basic arithmetic, string concatenation, list extension, and even real-world scenarios like score tracking. However, it's important to consider performance implications when dealing with large datasets to ensure optimal execution.
How += Works with Different Data Types
The `+=` operator, often referred to as the augmented assignment operator, is a versatile tool in programming that simplifies the process of updating variables by performing an operation and assigning the result back to the variable. This operator works seamlessly with various data types, making it a powerful and efficient way to write concise code. In this article, we will delve into how `+=` operates with different data types, exploring three key areas: **Integer and Float Operations**, **String Concatenation**, and **List and Tuple Extensions**. Understanding these different applications is crucial for writing effective and readable code. For instance, when dealing with numerical values, `+=` can be used to increment or accumulate values efficiently. Similarly, it can concatenate strings or extend lists and tuples in a straightforward manner. By examining each of these scenarios, developers can leverage the full potential of the `+=` operator to enhance their coding practices. Let's begin by examining how `+=` works with **Integer and Float Operations**, where we will see how this operator simplifies arithmetic operations and maintains precision across different numerical types.
Integer and Float Operations
When exploring the nuances of the `+=` operator in Python, it is crucial to understand how it interacts with different data types, particularly integers and floats. The `+=` operator is a shorthand for addition assignment, meaning it adds the value on its right to the variable on its left and assigns the result back to that variable. For integers, this operation is straightforward: `a += b` is equivalent to `a = a + b`, where both `a` and `b` are integers. For example, if `a` is initially 5 and `b` is 3, then after executing `a += b`, `a` will be 8. However, when dealing with floats, the behavior remains consistent but involves floating-point arithmetic. Floating-point numbers are represented as binary fractions, which can sometimes lead to minor precision issues due to rounding errors inherent in floating-point representation. Despite this, the `+=` operator still functions as expected: `a += b` where both `a` and `b` are floats will add them together and assign the result back to `a`. For instance, if `a` is initially 5.0 and `b` is 3.5, then after executing `a += b`, `a` will be 8.5. One of the key aspects to consider when using `+=` with mixed data types (i.e., combining integers and floats) is type coercion. In Python, when you add an integer to a float using `+=`, the integer is automatically converted to a float before the addition takes place. This ensures that the operation can proceed without type errors. For example, if `a` is initially 5 (an integer) and you execute `a += 3.5` (a float), Python will first convert `a` to a float (5.0), then perform the addition resulting in 8.5, which is then assigned back to `a`. Understanding these behaviors is essential for writing robust and predictable code in Python. It allows developers to leverage the flexibility of Python's dynamic typing while maintaining control over how different data types interact within their programs. Additionally, being aware of potential precision issues with floating-point numbers can help in designing algorithms that account for such nuances, ensuring accurate results even in complex numerical computations. In summary, the `+=` operator works seamlessly with both integers and floats in Python by performing addition and assignment in a manner that respects their respective arithmetic rules. Whether dealing solely with integers or combining them with floats, this operator simplifies code by encapsulating two operations into one concise statement, making it a powerful tool for any Python programmer.
String Concatenation
String concatenation is a fundamental operation in Python that allows you to combine multiple strings into a single string. When discussing how the `+=` operator works with different data types, understanding string concatenation is crucial. In Python, the `+=` operator can be used to concatenate strings by appending one string to another. For instance, if you have two variables `a` and `b` containing strings, using `a += b` will result in `a` being updated to contain the concatenated string of the original value of `a` followed by the value of `b`. One of the key aspects of string concatenation with `+=` is its efficiency and readability. Unlike other methods such as using the `+` operator repeatedly or employing the `join()` method, `+=` provides a concise way to build strings incrementally. This is particularly useful in loops where you might need to construct a large string from smaller parts. For example, if you are reading lines from a file and want to concatenate them into a single string, using `+=` simplifies the process significantly. Moreover, Python's dynamic typing allows for flexible use of the `+=` operator with various data types when dealing with strings. However, it's important to note that attempting to concatenate non-string data types directly will result in a TypeError. To avoid this, you must ensure that all operands are converted to strings before performing the concatenation. This can be done using methods like `str()` or f-strings (formatted string literals), which provide powerful tools for converting and formatting data into strings. For example: ```python a = "Hello" b = 42 a += str(b) # Converts b to a string before concatenation print(a) # Output: "Hello42" ``` In this example, `str(b)` converts the integer `42` into a string, allowing it to be concatenated with `"Hello"` without raising an error. Additionally, when working with different data types that need to be concatenated as strings, f-strings offer an elegant solution: ```python name = "Alice" age = 30 greeting = f"Hello, {name} You are {age} years old." print(greeting) # Output: "Hello, Alice You are 30 years old." ``` Here, f-strings automatically convert the variables `name` and `age` into their respective string representations within the formatted string. In summary, understanding how `+=` works with string concatenation in Python is essential for effective and efficient coding practices. By leveraging this operator along with appropriate type conversions and formatting tools like f-strings, developers can write clear and robust code that handles various data types seamlessly. This knowledge not only enhances code readability but also ensures that your programs operate correctly across diverse scenarios involving string manipulation.
List and Tuple Extensions
When exploring how the `+=` operator works in Python, it is crucial to understand its behavior with different data types, particularly with lists and tuples. Lists and tuples are fundamental data structures in Python, each with unique characteristics that influence how they interact with the `+=` operator. **Lists**: In Python, lists are mutable sequences, meaning they can be modified after creation. When you use the `+=` operator with a list, it behaves similarly to the `extend` method. For example, if you have two lists `list1` and `list2`, using `list1 += list2` will append all elements of `list2` to `list1`. This operation modifies the original list by adding new elements at the end. Here’s an example: ```python list1 = [1, 2, 3] list2 = [4, 5, 6] list1 += list2 print(list1) # Output: [1, 2, 3, 4, 5, 6] ``` This behavior is efficient because it avoids creating a new list and instead modifies the existing one in place. **Tuples**: Tuples, on the other hand, are immutable sequences. Once a tuple is created, its contents cannot be changed. When you attempt to use the `+=` operator with a tuple, it does not modify the original tuple but instead creates a new tuple that is the concatenation of the original tuple and the other iterable. Here’s an example: ```python tuple1 = (1, 2, 3) tuple2 = (4, 5, 6) tuple1 += tuple2 print(tuple1) # Output: (1, 2, 3, 4, 5, 6) ``` In this case, `tuple1` is reassigned to point to a new tuple that results from concatenating `tuple1` and `tuple2`. The original `tuple1` remains unchanged until it is reassigned. **Key Differences**: - **Mutability**: Lists are mutable; tuples are immutable. - **In-place Modification**: Using `+=` with lists modifies them in place; using `+=` with tuples creates a new tuple. - **Performance**: Modifying lists in place can be more efficient than creating new tuples. Understanding these differences is essential for writing efficient and correct code when working with lists and tuples in Python. By knowing how the `+=` operator behaves with these data structures, you can leverage their unique properties to achieve your programming goals effectively. In summary, while both lists and tuples support concatenation via the `+=` operator, their immutability and mutability characteristics lead to distinct outcomes. Lists are extended in place, enhancing performance by avoiding unnecessary object creation, whereas tuples result in new objects being created each time they are concatenated. This nuanced understanding helps developers make informed decisions about when to use each data structure based on their specific needs.
Best Practices and Pitfalls with += in Python
When working with the `+=` operator in Python, it is crucial to understand the best practices and potential pitfalls to ensure efficient and error-free code. This article delves into three key areas: **Performance Considerations**, **Avoiding Common Mistakes**, and **Advanced Usage Scenarios**. Understanding these aspects can significantly enhance your coding skills and help you avoid common traps. Starting with **Performance Considerations**, it is essential to recognize how the `+=` operator behaves differently depending on the type of object being modified. For instance, when used with mutable objects like lists, `+=` can lead to unexpected performance issues if not managed properly. On the other hand, using `+=` with immutable objects like strings or integers involves creating new objects, which can impact memory usage and execution speed. By **Avoiding Common Mistakes**, developers can steer clear of bugs that arise from misunderstandings about how `+=` interacts with different data types. For example, using `+=` on a list within a loop can inadvertently modify the original list if it is passed by reference, leading to unintended side effects. Finally, exploring **Advanced Usage Scenarios** reveals how `+=` can be leveraged in creative ways to simplify complex operations and improve code readability. However, these advanced uses also come with their own set of challenges that must be carefully navigated. In the following sections, we will dive deeper into each of these areas, beginning with **Performance Considerations** to ensure that your use of `+=` is both efficient and effective.
Performance Considerations
When discussing the use of the `+=` operator in Python, it is crucial to consider performance implications, as they can significantly impact the efficiency and scalability of your code. The `+=` operator, while convenient for concatenating strings or augmenting values, can lead to performance bottlenecks if not used judiciously. For instance, when concatenating strings using `+=`, Python creates a new string object each time the operation is performed. This can result in quadratic time complexity for large numbers of concatenations, leading to substantial performance degradation. Instead, consider using a list to accumulate strings and then joining them at once using the `join()` method, which is much more efficient. In numerical operations, `+=` generally performs well because it modifies the existing object in place for mutable types like lists and dictionaries. However, for immutable types such as integers and floats, a new object is created each time, which can still lead to memory allocation overheads if performed repeatedly. Another performance consideration is the context in which `+=` is used. In loops, especially those with many iterations, using `+=` on mutable objects can be efficient but should be balanced against other operations that might introduce unnecessary overhead. For example, if you are appending elements to a list within a loop using `+=`, ensure that other operations within the loop do not negate the benefits of using this operator. Additionally, understanding how Python's memory management works is essential. Frequent use of `+=` on large objects can lead to increased memory usage and potential garbage collection pauses, affecting overall system performance. Best practices include avoiding excessive use of `+=` for string concatenation and opting for more efficient methods like list accumulation followed by joining. For numerical operations, ensuring that you are working with mutable types where possible can help minimize object creation overheads. In summary, while the `+=` operator is a powerful tool in Python, its performance implications must be carefully considered to avoid unintended bottlenecks. By understanding how this operator interacts with different data types and using it judiciously within your code, you can write more efficient and scalable programs. This mindful approach not only enhances performance but also contributes to better coding practices overall.
Avoiding Common Mistakes
When working with the `+=` operator in Python, it is crucial to avoid common mistakes that can lead to unexpected behavior or errors. One of the most frequent pitfalls is misunderstanding the operator's behavior with different data types. For instance, when using `+=` with lists, it does not perform element-wise addition but instead extends the list with the elements of another list. This can be confusing if you are accustomed to other languages where similar operators behave differently. For example, `a = [1, 2]; b = [3, 4]; a += b` will result in `a` becoming `[1, 2, 3, 4]`, not `[4, 6]`. Another common mistake is using `+=` with mutable objects in a way that modifies the original object unexpectedly. When you use `+=` on a mutable object like a list or dictionary and assign it to another variable, both variables will point to the same modified object. For example, `a = [1, 2]; b = a; a += [3, 4]` will make both `a` and `b` equal to `[1, 2, 3, 4]`. This can lead to unintended side effects if not managed carefully. Additionally, it's important to be aware of the difference between `+=` and other operators like `*=` when dealing with strings and lists. While `*=` repeats the string or list a specified number of times, `+=` concatenates or extends them. For instance, `s = 'hello'; s *= 2` results in `'hellohello'`, whereas `s = 'hello'; s += 'world'` results in `'helloworld'`. Moreover, when working with numeric types such as integers and floats, ensure that you are not mixing types inadvertently. For example, if you start with an integer and then use `+=` with a float value, the result will be a float which might change your expected outcome. To avoid these mistakes effectively: 1. **Understand Data Types**: Be clear about how `+=` behaves with different data types such as lists, strings, integers, and floats. 2. **Use Immutable Objects Wisely**: When working with mutable objects like lists or dictionaries assigned to multiple variables, ensure you understand how modifications affect all references. 3. **Test Your Code**: Always test your code thoroughly especially when using complex operations involving multiple variables. 4. **Read Documentation**: Refer to Python documentation for specific behaviors of operators like `+=` to avoid assumptions based on other languages. By being mindful of these potential pitfalls and following best practices such as clear understanding of data types and careful handling of mutable objects, you can leverage the power of the `+=` operator effectively in your Python code without encountering unexpected issues. This approach not only enhances your coding skills but also ensures robustness and reliability in your programs.
Advanced Usage Scenarios
When delving into advanced usage scenarios involving the `+=` operator in Python, it is crucial to understand its versatility and potential pitfalls. One of the most common advanced uses of `+=` is in conjunction with mutable objects such as lists and dictionaries. For instance, when you use `+=` with a list, it behaves similarly to the `extend` method, allowing you to append multiple elements at once. However, this can lead to unexpected behavior if not handled carefully. For example, if you have a list of lists and use `+=` to append another list, it will extend the outer list with the inner list's elements rather than appending the inner list itself. Another advanced scenario involves using `+=` with custom classes that implement the `__iadd__` method. This method allows you to define how the `+=` operator should behave for your custom objects. However, it's important to ensure that this method modifies the object in place and returns `self` to maintain consistency with built-in types. Failure to do so can result in confusing behavior where the object appears to be modified but actually isn't. In the context of best practices, it's essential to be mindful of the type of object you are working with. For immutable types like strings and tuples, `+=` creates a new object each time it is used, which can be inefficient if performed in a loop. Instead, consider using a mutable type like a list or a string buffer (such as `io.StringIO`) and then converting it back to the desired type at the end. Moreover, when using `+=` in loops or recursive functions, ensure that you are not inadvertently creating unintended side effects. For example, if you are using `+=` to accumulate results in a loop and the variable is defined outside the loop's scope, it will retain its value across iterations unless explicitly reset. Pitfalls often arise from misunderstandings about how `+=` interacts with different data types and scopes. A common mistake is assuming that `+=` always modifies the original object; however, this is only true for mutable objects. With immutable objects like integers or strings, `+=` reassigns the variable to a new object rather than modifying the existing one. To avoid these pitfalls and ensure best practices are followed: 1. **Understand Mutability**: Be clear whether you are working with mutable or immutable objects. 2. **Custom Classes**: Ensure that custom classes correctly implement `__iadd__` if you intend to use them with `+=`. 3. **Efficiency**: Use mutable types when performing repeated concatenations or accumulations. 4. **Scope Awareness**: Be aware of variable scope when using `+=` in loops or recursive functions. By understanding these advanced usage scenarios and adhering to best practices, you can leverage the power of the `+=` operator effectively while avoiding common pitfalls that can lead to bugs and inefficiencies in your code.